SpringBoot 基础篇:依赖管理
SpringBoot 基础篇:依赖管理
父项目做依赖管理
在 Maven 中,一般我们引入夫项目都是为了做依赖管理
参考 《Maven 听课笔记.docx》中的
Maven 管理多模块应用
小节,从这个小节中我们可以直到:
子模块会无条件继承父模块的
<properties>
设置子模块会无条件继承(引入)父模块
<dependencies>
中的所有依赖。子模块会无条件继承(引入)父模块
<plugins>
中的所有依赖。子模块不会自动引入父模块 POM 中
<dependencyManagement>
中的依赖,在其需要的情况下,可以仅通过 groupId、artifactId(不需要 version)来引入(这就是声明式依赖),版本默认为父模块 POM 中<dependencyManagement>
中的版本,子模块不会自动引入父模块 POM 中
<pluginManagement>
的插件,在其需要的情况下,可以仅通过 groupId、artifactId(不需要 version)来引入(这就是声明式依赖),版本默认为父模块 POM 中<pluginManagement>
中的版本
我们在新建 SpringBoot 应用的时候,pom 文件中会引入父项目,注意,<version>2.3.4.RELEASE</version>
是我们在当前应用的 POM 文件中唯一指定的版本信息,指定了我们引入的 SpringBoot 的大版本。
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.9.RELEASE</version>
</parent>
看其具体的内容,我们发现,spring-boot-starter-parent
主要是设置 <pluginManagement>
,不过这些插件同样没有设置版本,可以想象这些插件应该都是从其父项目继承
同时我们还发现
<resources>
<resource>
<directory>${basedir}/src/main/resources</directory>
<!-- 支持插值 -->
<filtering>true</filtering>
<!-- src/main/resources 路径下任意层路径下的以application开头的,以yml、yaml、properties结尾的文件,都会被识别为有效的配置文件 -->
<!-- 但是默认只有文件名为application的生效 -->
<includes>
<include>**/application*.yml</include>
<include>**/application*.yaml</include>
<include>**/application*.properties</include>
</includes>
</resource>
<resource>
<directory>${basedir}/src/main/resources</directory>
<excludes>
<exclude>**/application*.yml</exclude>
<exclude>**/application*.yaml</exclude>
<exclude>**/application*.properties</exclude>
</excludes>
</resource>
</resources>
默认不处理 xml
配置。
继续看 spring-boot-starter-parent
的父项目 spring-boot-dependencies
:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.3.9.RELEASE</version>
</parent>
spring-boot-dependencies
包含了几乎所有的常用 jar 包的版本信息:包含大量的 <properties>
、<dependencyManagement>
和 <pluginManagement>
,其中 <dependencyManagement>
和 <pluginManagement>
的版本信息基本上都是 <properties>
中的元素,所以如果你想在 SpringBoot 应用中用别的版本的依赖或者插件,直接在当前应用的 POM 文件中覆盖相应的 <properties>
属性即可。
举个例子,我想引入 MySQL 驱动,这个依赖在 spring-boot-dependencies
的 <dependencyManagement>
中存在,默认版本是 <mysql.version>8.0.21</mysql.version>
,因此当前应用不需要指定版本号
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
然后我们发现 Maven 引入了相关依赖,
此时我们修改版本,
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<mysql.version>6.0.6</mysql.version>
</properties>
再次导入之后,发现版本变为我们指定的版本。
当然我们也可以直接在引入依赖的时候绑定,但是这样不够优雅。
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>6.0.6</version>
</dependency>
IDEA 的智能操作
当我们在 POM 文件中想要添加 spring-boot-dependencies
中 <dependencyManagement>
管理的依赖的时候,直接右键或者 Alt+Insert
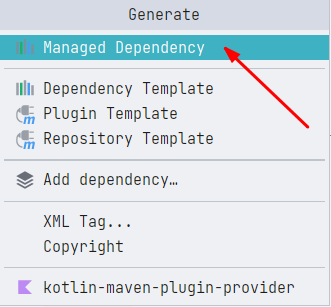
选择 Managed Dependency
就可以看到当前 POM 中所有的被管理的依赖
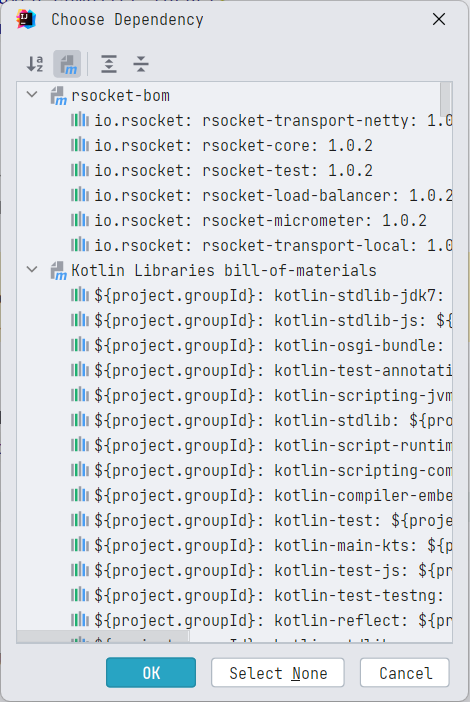
选择你想要的依赖点击 OK 即可导入
开发导入 starter 场景启动器
官方文档地址,其中包含了所有的 starter 的列表,即 Spring Boot 支持的所有场景。常见的 starter 有
-
spring-boot-starter-web
: 使用 Spring MVC 构建 web(包括 RESTful) 应用程序的启动器。使用 Tomcat 作为默认的嵌入式容器。做 web 必引入 -
spring-boot-starter-thymeleaf
:使用 Thymeleaf 视图构建 MVC web 应用程序的启动器,也是做 web 必备。 -
spring-boot-starter-json
:读写 JSON 的启动器,做 Web 必备 -
spring-boot-starter-aop
:使用 Spring AOP 和 AspectJ 进行面向切面编程的启动器 -
spring-boot-starter-test
:使用 JUnit、Hamcrest 和 Mockito 库的测试 Spring Boot 应用程序的启动器 -
spring-boot-starter-quartz
:使用 Quartz 调度器的启动器 -
spring-boot-starter-validation
:使用 Hibernate Validator 进行 Java Bean 验证的启动器 -
spring-boot-starter-data-redis
:通过 Spring data Redis 和 Lettuce 客户端使用 Redis 键值数据存储的启动器 -
spring-boot-starter-data-neo4j
:使用 Neo4j 图形数据库和 Spring Data Neo4j 的启动器 -
spring-boot-starter-data-jpa
:在通过 Hibernate 使用 Spring Data JPA 的启动器 -
spring-boot-starter-jdbc
:配合 HikariCP 连接池使用 JDBC 的启动器 -
spring-boot-starter-data-jdbc
:使用 Spring Data JDBC 的启动器 -
spring-boot-starter-batch
:使用 Spring Batch 的启动器
命名格式是 spring-boot-starter-*
一个 *
代表就某种场景。只要引入 starter,这个场景的所有常规需要的依赖我们都自动引入,因为每一个 starter 下都有很多依赖,根据传递依赖,当前应用依赖了 starter,那么 starter 的依赖,在当前应用中也会引用,
这实际上也是 maven 的传递依赖的特性,当然,传递依赖会有冲突,这个 starter0 依赖了 A 的一个版本 a0,另一个 starter1 依赖了 A 的另一个版本 a1,当前应用同时依赖这 starter0 和 starter1 的时候,A 就会有版本冲突,这个问题,实际上各个 starter 以及协调好了,不会出现冲突,这也是 SpringBoot 制作 starter 解决的问题之一,消除版本冲突。
除了官方 Starter,还有第三方 starter,命名格式为:*-spring-boot-starter
,*
就代表第三方机构的名称。社区中的第三方 Starter
所有的 spring boot starter 都有一个依赖,注意是依赖不是父项目:spring-boot-start
,代表所有场景中的基本的场景所需要的依赖,spring-boot-start
是一个核心启动器,包括对自动的配置支持、日志记录和 YAML。这个我们后面会了解到
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.3.9.RELEASE</version>
<scope>compile</scope>
</dependency>
之前在使用 SpringMVC 的时候,每次创建项目都要引入各种依赖,比如处理 JSON 的,进行验证的 Validation,还要控制版本,确实很烦,有了 starter,就非常方便了
无需关注版本号,自动版本仲裁
-
引入依赖默认都可以不写版本
-
引入非版本仲裁的 jar,要写版本号。
怎么判断呢,就看这个依赖在 spring-boot-dependencies
中存不存在,存在的话,说明其版本 SpringBoot 就已经帮我们管理了,否则,就需要我们自己指定版本。
可以修改默认版本号
查看 父项目做依赖管理
小节